You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Copy file name to clipboardExpand all lines: README.md
+168-19
Original file line number
Diff line number
Diff line change
@@ -6,7 +6,7 @@ It requires an appropriate **Python module to be installed separately** - depend
6
6
The library consists of some keywords designed to perform different checks on your database.
7
7
Here you can find the [keyword docs](http://marketsquare.github.io/Robotframework-Database-Library/).
8
8
9
-
Wath the [talk at Robocon 2024 about the Database Library update](https://youtu.be/A96NTUps8sU)
9
+
Wath the [talk at Robocon 2024 about the Database Library update](https://youtu.be/A96NTUps8sU).
10
10
11
11
[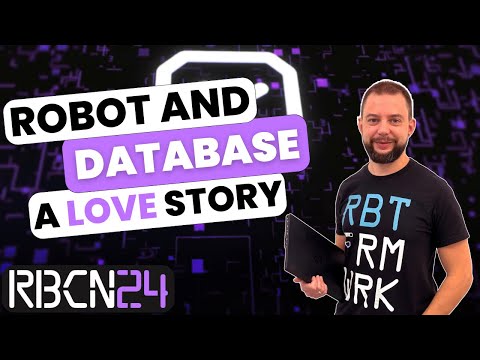](https://youtu.be/A96NTUps8sU)
12
12
@@ -18,8 +18,7 @@ Wath the [talk at Robocon 2024 about the Database Library update](https://youtu.
18
18
```
19
19
pip install robotframework-databaselibrary
20
20
```
21
-
# Usage examples
22
-
## Basic usage
21
+
# Basic usage examples
23
22
```RobotFramework
24
23
*** Settings ***
25
24
Library DatabaseLibrary
@@ -29,25 +28,40 @@ Test Setup Connect To My Oracle DB
29
28
Connect To My Oracle DB
30
29
Connect To Database
31
30
... oracledb
32
-
... dbName=db
33
-
... dbUsername=my_user
34
-
... dbPassword=my_pass
35
-
... dbHost=127.0.0.1
36
-
... dbPort=1521
31
+
... db_name=db
32
+
... db_user=my_user
33
+
... db_password=my_pass
34
+
... db_host=127.0.0.1
35
+
... db_port=1521
37
36
38
37
*** Test Cases ***
38
+
Get All Names
39
+
${Rows}= Query select FIRST_NAME, LAST_NAME from person
40
+
Should Be Equal ${Rows}[0][0] Franz Allan
41
+
Should Be Equal ${Rows}[0][1] See
42
+
Should Be Equal ${Rows}[1][0] Jerry
43
+
Should Be Equal ${Rows}[1][1] Schneider
44
+
39
45
Person Table Contains Expected Records
40
-
${output}= Query select LAST_NAME from person
41
-
Length Should Be ${output} 2
42
-
Should Be Equal ${output}[0][0] See
43
-
Should Be Equal ${output}[1][0] Schneider
46
+
${sql}= Catenate select LAST_NAME from person
47
+
Check Query Result ${sql} contains See
48
+
Check Query Result ${sql} equals Schneider row=1
49
+
50
+
Wait Until Table Gets New Record
51
+
${sql}= Catenate select LAST_NAME from person
52
+
Check Row Count ${sql} > 2 retry_timeout=5s
44
53
45
54
Person Table Contains No Joe
46
55
${sql}= Catenate SELECT id FROM person
47
-
... WHERE FIRST_NAME= 'Joe'
48
-
Check If Not Exists In Database ${sql}
56
+
... WHERE FIRST_NAME= 'Joe'
57
+
Check Row Count ${sql} == 0
49
58
```
50
-
## Handling multiple database connections
59
+
See more examples in the folder `tests`.
60
+
61
+
# Handling multiple database connections
62
+
The library can handle multiple connections to different databases using *aliases*.
63
+
An alias is set while creating a connection and can be passed to library keywords in a corresponding argument.
64
+
## Example
51
65
```RobotFramework
52
66
*** Settings ***
53
67
Library DatabaseLibrary
@@ -56,9 +70,21 @@ Test Teardown Disconnect From All Databases
56
70
57
71
*** Keywords ***
58
72
Connect To All Databases
59
-
Connect To Database psycopg2 db db_user pass 127.0.0.1 5432
73
+
Connect To Database
74
+
... psycopg2
75
+
... db_name=db
76
+
... db_user=db_user
77
+
... db_password=pass
78
+
... db_host=127.0.0.1
79
+
... db_port=5432
60
80
... alias=postgres
61
-
Connect To Database pymysql db db_user pass 127.0.0.1 3306
81
+
Connect To Database
82
+
... pymysql
83
+
... db_name=db
84
+
... db_user=db_user
85
+
... db_password=pass
86
+
... db_host=127.0.0.1
87
+
... db_port=3306
62
88
... alias=mysql
63
89
64
90
*** Test Cases ***
@@ -73,7 +99,130 @@ Switching Default Alias
73
99
Execute Sql String drop table XYZ
74
100
```
75
101
76
-
See more examples in the folder `tests`.
102
+
103
+
104
+
# Using configuration file
105
+
The `Connect To Database` keyword allows providing the connection parameters in two ways:
106
+
- As keyword arguments
107
+
- In a configuration file - a simple list of _key=value_ pairs, set inside an _alias_ section.
108
+
109
+
You can use only one way or you can combine them:
110
+
- The keyword arguments are taken by default
111
+
- If no keyword argument is provided, a parameter value is searched in the config file
112
+
113
+
Along with commonly used connection parameters, named exactly as keyword arguments, a config file
114
+
can contain any other DB module specific parameters as key/value pairs.
115
+
If same custom parameter is provided both as a keyword argument *and* in config file,
116
+
the *keyword argument value takes precedence*.
117
+
118
+
The path to the config file is set by default to `./resources/db.cfg`.
119
+
You can change it using an according parameter in the `Connect To Database` keyword.
120
+
121
+
A config file *must* contain at least one section name -
122
+
the connection alias, if used (see [Handling multiple database connections](#handling-multiple-database-connections)), or
123
+
`[default]` if no aliases are used.
124
+
125
+
## Config file examples
126
+
### Config file with default alias (equal to using no aliases at all)
127
+
```
128
+
[default]
129
+
db_module=psycopg2
130
+
db_name=yourdbname
131
+
db_user=yourusername
132
+
db_password=yourpassword
133
+
db_host=yourhost
134
+
db_port=yourport
135
+
```
136
+
### Config file with a specific alias
137
+
```
138
+
[myoracle]
139
+
db_module=oracledb
140
+
db_name=yourdbname
141
+
db_user=yourusername
142
+
db_password=yourpassword
143
+
db_host=yourhost
144
+
db_port=yourport
145
+
```
146
+
147
+
### Config file with some params only
148
+
```
149
+
[default]
150
+
db_password=mysecret
151
+
```
152
+
### Config file with some custom DB module specific params
153
+
```
154
+
[default]
155
+
my_custom_param=value
156
+
```
157
+
158
+
# Inline assertions
159
+
Keywords, that accept arguments ``assertion_operator`` and ``expected_value``,
160
+
perform a check according to the specified condition - using the [Assertion Engine](https://github.com/MarketSquare/AssertionEngine).
161
+
162
+
## Examples
163
+
```RobotFramework
164
+
Check Row Count SELECT id FROM person == 2
165
+
Check Query Result SELECT first_name FROM person contains Allan
166
+
```
167
+
168
+
# Retry mechanism
169
+
Assertion keywords, that accept arguments ``retry_timeout`` and ``retry_pause``, support waiting for assertion to pass.
170
+
171
+
Setting the ``retry_timeout`` argument enables the mechanism -
172
+
in this case the SQL request and the assertion are executed in a loop,
173
+
until the assertion is passed or the ``retry_timeout`` is reached.
174
+
The pause between the loop iterations is set using the ``retry_pause`` argument.
175
+
176
+
The argument values are set in [Robot Framework time format](http://robotframework.org/robotframework/latest/RobotFrameworkUserGuide.html#time-format) - e.g. ``5 seconds``.
177
+
178
+
The retry mechanism is disabled by default - ``retry_timeout`` is set to ``0``.
- Both thick and thin client modes are supported - you can select one using the `driverMode` parameter.
235
+
- Both thick and thin client modes are supported - you can select one using the `oracle_driver_mode` parameter.
87
236
- However, due to current limitations of the oracledb module, **it's not possible to switch between thick and thin modes during a test execution session** - even in different suites.
0 commit comments